Doing things a little more properly part 2
In the last post, we made a new sprite using the SDK’s sprite abilities. In this post, let’s make our cat sprite in the same way.
This is very very simple. Here’s the code for our cat sprite. This is still not right, we should be using the SDK animation system but let’s do that a little later.
class("CatSprite").extends(playdate.graphics.sprite)
local gfx <const> = playdate.graphics
local catSheet = gfx.imagetable.new("images/cat_sheet")
assert(catSheet, "Failed to load cat_sheet")
function CatSprite:init(x, y)
CatSprite.super.init(self)
local image = catSheet:getImage(1) -- Get the first image
self:setImage(image)
self:moveTo(x, y)
end
count = 1
function CatSprite:update()
count += 1
if count > 20 then
count = 1
end
image_number = math.ceil(count / 5)
local image = catSheet:getImage(image_number)
self:setImage(image)
end
local sprite = CatSprite(200, 150)
sprite:add()
We start of with using the SDK class system to extend the playdate sprite class. Note that we don’t do any imports here, it appears everything which is imported in the main.lua appears here as well. Not sure if that is a feature of Lua or a feature of the SDK.
Lines 8 to 13 define our cat sprite init method, which get called when we create the sprite. Line 9 calls the superclass init method otherwise we’d lose all our SDK goodness. This init method takes two parameters, x and y, which defines where the sprite will be placed. Now we set our first image in the image table as the first image of the sprite and moves to whatever position we give it.
Lines 15 to 26 are taken from the old update routine in main.lua and doing the same thing. Changing the image every five frames and looping through the four images of the image table. This is a little inefficient as we’re updating the image even if it hasn’t changed which we don’t need to do but we’re a long way from worrying about efficiency.
We then create the sprite and add it (otherwise it won’t be handled by the sprite mechanism.
Our main.lua file is now pristeen!
import "Corelibs/graphics"
import "Corelibs/sprites"
import "Corelibs/object"
import "cat"
import "meow"
local gfx <const> = playdate.graphics
function playdate.update()
gfx.clear()
gfx.sprite.update()
end
Oh, OK – let’s do one last thing. Rotate the text image. Also trivial. Just add a new update method to the MeowSprite which looks like this:
rotation = 0
function MeowSprite:update()
rotation += 10
self:setRotation(rotation)
self:setImage(image)
end
Resulting in
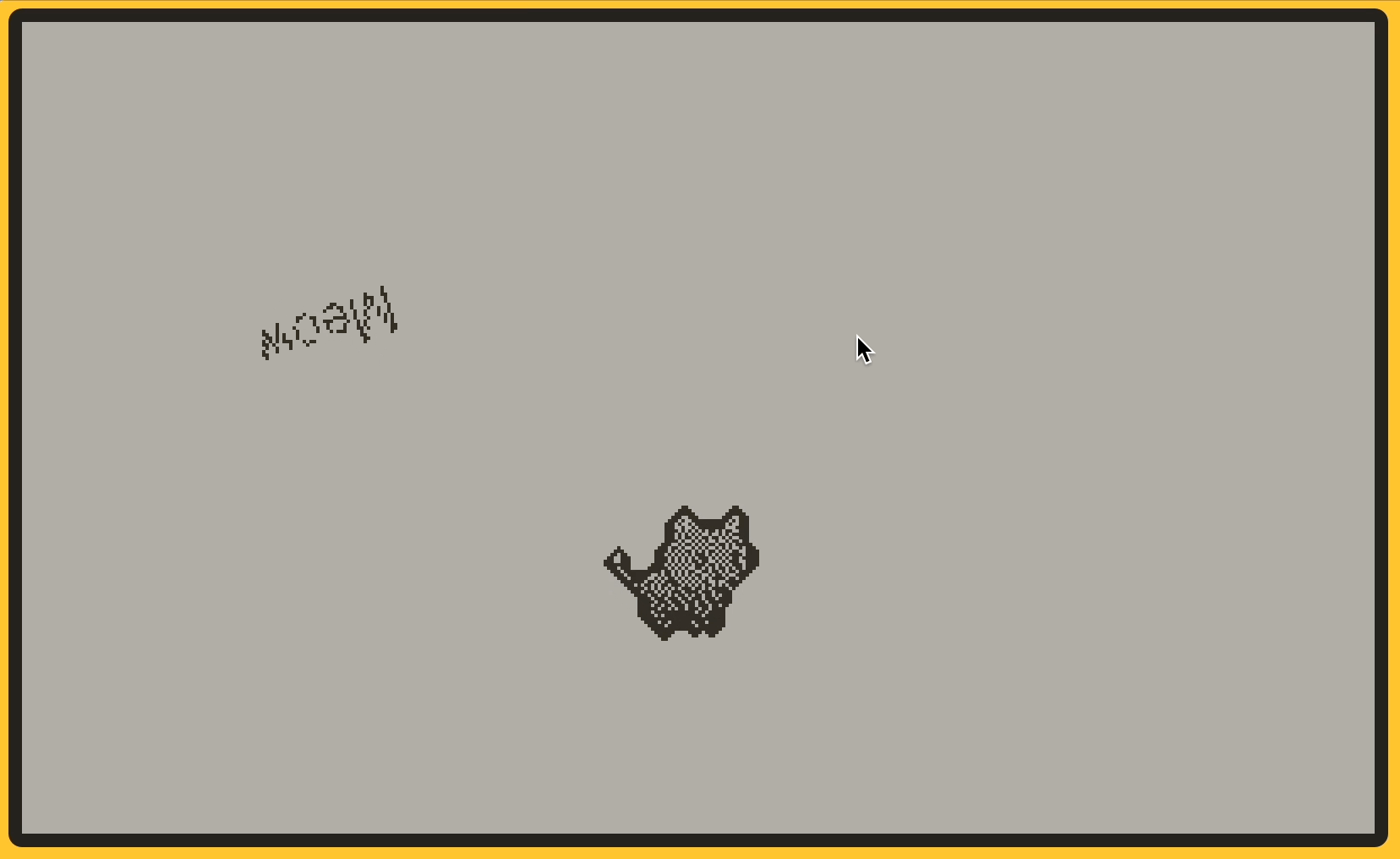