Doing things a little more “properly” part 1
In my last post I got an animation of a cat going but it was an ugly method. I’m an object oriented programmer and will struggle not to be. Lua has strategies for handling OOP although it doesn’t have OOP baked in from what I can see. More importantly for me, the Playdate SDK provides OOP methods as well.
So let’s try and get an OOP sprite running and this time I’m going to make my life a little easier by focussing on making a text based sprite which moves around the screen. Goals are:
- Make a sprite using the SDK class system
- Move it around the screen
- Detect if we hit the cat
- Change the word used by the sprite randomly
Making an SDK Sprite
Once again, I am still learning Lua and making a lot of silly mistakes. One I keep making is using the . operator instead of the : operator.
function MeowSprite:init(x, y)
MeowSprite.super.init(self)
self.setImage(image)
self:moveTo(x,y)
end
Which results in the following error
meow.lua:15: bad argument #1 to 'setImage' (playdate.graphics.sprite expected, got playdate.graphics.image)
I was confused for quite a while (and blind) thinking that setImage wanted a sprite and not an image which would be nuts. The error is simple once you understand the mistake. setImage is actually expecting two parameters, self (which is a sprite) and the image. Self is passed automatically when using : and therefore.
function MeowSprite:init(x, y)
MeowSprite.super.init(self)
-- This is stupid but works :)
self.setImage(self, image)
self.moveTo(self, x,y)
end
The ‘:’ notation is a shortcut for the above. Of course we should be doing:
function MeowSprite:init(x, y)
MeowSprite.super.init(self)
self:setImage(image)
self:moveTo(x,y)
end
So at the end of that detour our sprite file meow.lua
contains the following code:
class("MeowSprite").extends(playdate.graphics.sprite)
local image = playdate.graphics.imageWithText("Meow", 40, 40)
assert(image)
function MeowSprite:init(x, y)
MeowSprite.super.init(self)
self:setImage(image)
self:moveTo(x,y)
end
local sprite = MeowSprite(90,90)
sprite:add()
Which results in …
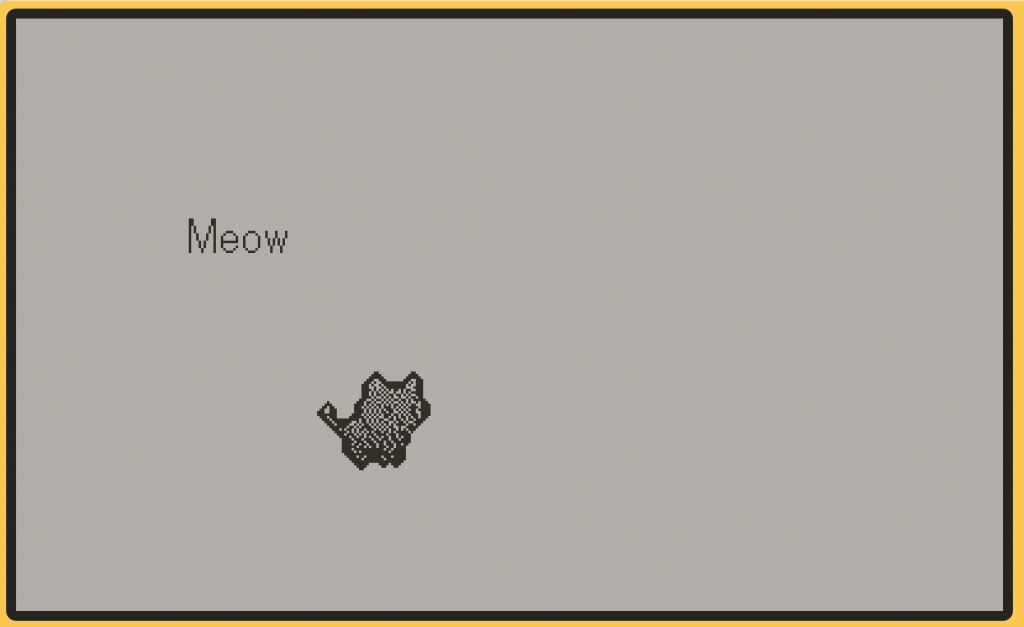
Exciting stuff !
I should note also that in our main.lua file I needed to make a call to the sprite update method within the playdate.update method to draw this sprite (and other sprites we define in this method).
playdate.graphics.sprite.update()